はじめに
今回から四節リンク機構をProcessingとFusion360で作っていきたいと思います。
四節リンク機構は、機構の各節の長さを変更することで以下の特徴を持つ機構になります。
- てこクランク機構
- 両クランク機構
- 両てこ機構
今回は、てこクランク機構を作成したいと思います。
てこクランク機構とは?
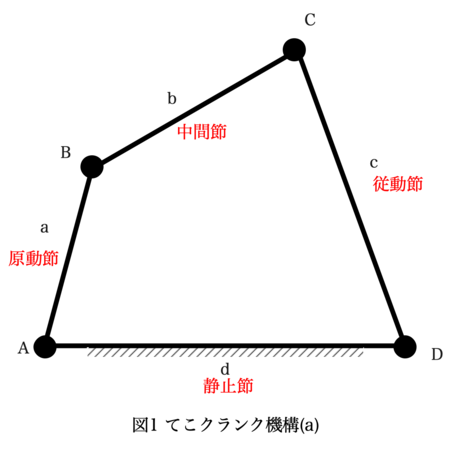
図1のように、リンクd(静止節)を固定し、リンクa(原動節)を1回転させるとリンクc(従動節)が揺動運動する機構をてこクランク機構といいます。
最短リンクをaとし、原動節aが1回転する条件は、グラフホフの定理より以下の3角式を同時に満たす場合です。
a+b ≤ c+d , a+c ≤ b+d , a+d ≤ b+c
点Bと点Dの距離sと角度を求める
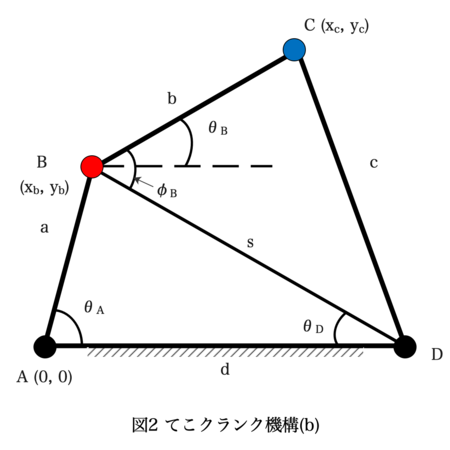
図2より、点Bと点Cの距離sと角度を求めましょう。
点Bと点Cの距離sは、余弦定理より
\[s=\sqrt{a^2+b^2-2ab\cos\theta_A}\]
\(\angle\)ADBの大きさ\(\theta_D\)は、点Bより垂直に引いた線を\(a\sin\theta_A\)とし、正弦定理より
\[\theta_D=\sin^{-1}(\frac{a\sin\theta_A}{s})\]
\(\angle\)CBDの大きさ\(\phi_B\)は、余弦定理より
\[\phi_B=\cos^{-1}(\frac{b^2+s^2-c^2}{2bs})\]
節bと水平軸とのなす角\(\theta_B\)は、平行線の錯覚が等しいため、\(\theta_B=\phi_B-\theta_D\)となります。
点B\((x_b,y_b)\)と点C\((x_c,y_c)\)の位置を求める
点Aを原点(0,0)としたとき、点Bの位置\((x_b,y_b)\)は
\begin{cases}
x_b=a\cos\theta_A \\
y_b=a\sin\theta_A
\end{cases}
よって、点Cの位置\((x_c,y_c)\)は
\begin{cases}
x_c=x_b+b\cos\theta_B \\
y_c=y_b+b\sin\theta_B
\end{cases}となります。
これらを用いてProcessingで表現していきましょう。
Processingでてこクランク機構
メインタブのコードは以下になります。
Processingではy軸は下方向なので、\(\phi_B\)をマイナスにします。
PGraphics pg1, pg2;
PVector Dot_A = new PVector(130, 300); //点Aを定義
PVector Dot_D = new PVector(390, 360); //点Dを定義
PVector Dot_B;
PVector Dot_C;
float theta_A = 0; //θAを初期化
float theta_B, theta_D, phi_B;
float r = 15;
float s;
void setup() {
size(500, 500);
background(255);
//PGraphicsを生成
pg1 = createGraphics(500, 500);
pg2 = createGraphics(500, 500);
}
void draw() {
background(255);
update(100, 280, 200); //辺a=100,b=280,c=200
display();
//Keyを押したら表示
if (showDriver) Driver();
if (showFollower) Follower();
if (showLine_s) Line_s();
}
void update(float a, float b, float c) {
//点Bの位置
float x_b = 130 + a * cos(theta_A);
float y_b = 300 + a * sin(theta_A);
Dot_B = new PVector(x_b, y_b);
s = dist(Dot_B.x, Dot_B.y, Dot_D.x, Dot_D.y); //辺BDを計算
theta_D = asin((a * sin(theta_A)) / s); //θDを計算
phi_B = -acos((sq(b)+sq(s)-sq(c)) / (2 * b * s)); //φBを計算
theta_B = phi_B - theta_D; //θBを計算
//点Cの位置
float x_c = Dot_B.x + b * cos(theta_B);
float y_c = Dot_B.y + b * sin(theta_B);
Dot_C = new PVector(x_c, y_c);
theta_A += 0.03; //θAを更新して回転
}
void display() {
//リンクを生成
stroke(0);
strokeWeight(2);
line(Dot_A.x, Dot_A.y, Dot_B.x, Dot_B.y);
line(Dot_B.x, Dot_B.y, Dot_C.x, Dot_C.y);
line(Dot_C.x, Dot_C.y, Dot_D.x, Dot_D.y);
//各点を生成
noStroke();
fill(0);
ellipse(Dot_A.x, Dot_A.y, r, r);
ellipse(Dot_D.x, Dot_D.y, r, r);
fill(209, 41, 26);
ellipse(Dot_B.x, Dot_B.y, r, r);
fill(0, 40, 205);
ellipse(Dot_C.x, Dot_C.y, r, r);
}
点Bと点Dの距離sをProcessingで計算するのは、dist関数を使いました。
s = dist(Dot_B.x, Dot_B.y, Dot_D.x, Dot_D.y); //辺BDを計算
以下は、キーボードのc,b,sを押すと運動の軌跡と距離sの伸縮を描くコードです。
別タブにコードを書きましょう。
draw関数内にbackground(255)があるため、軌跡を消さないようにPGraphicsを使いました。
boolean showDriver = false;
boolean showFollower = false;
boolean showLine_s = false;
//原動節の軌跡
void Driver() {
pg1.beginDraw();
pg1.noStroke();
pg1.fill(209, 41, 26);
pg1.ellipse(Dot_B.x, Dot_B.y, 5, 5);
pg1.endDraw();
image(pg1, 0, 0);
}
//従動節の軌跡
void Follower() {
pg2.beginDraw();
pg2.noStroke();
pg2.fill(0, 40, 205);
pg2.ellipse(Dot_C.x, Dot_C.y, 5, 5);
pg2.endDraw();
image(pg2, 0, 0);
}
void Line_s() {
stroke(0);
line(Dot_B.x, Dot_B.y, Dot_D.x, Dot_D.y);
}
void keyPressed() {
if (key == 'b') {
showDriver = !showDriver;
if (!showDriver) showDriver = false;
} else if (key == 'c') {
showFollower = !showFollower;
if (!showFollower) showFollower = false;
} else if (key == 's') {
showLine_s = !showLine_s;
if (!showLine_s) showLine_s = false;
}
}
アニメーション
実際の動作は以下のようになります。
おまけ:Fusion360でてこクランク機構
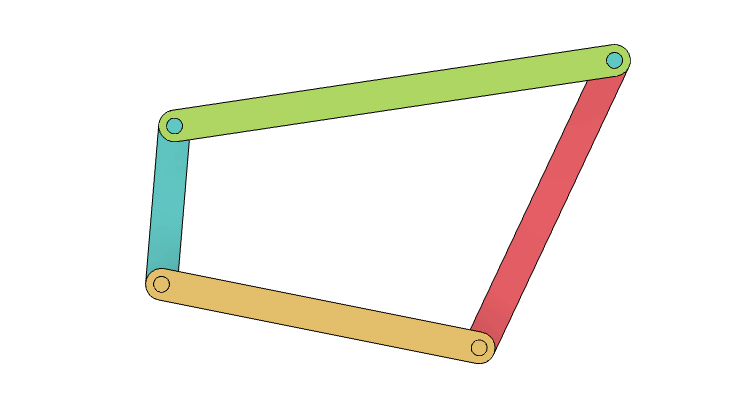
てこクランク機構の動きを理解するために、Fusion360でてこクランク機構のアニメーションをしてみました。
参考文献
・リンク機構 出典:機械設計法 塚田忠夫等 共著 森北出版株式会社
http://www.ecs.shimane-u.ac.jp/~shutingli/MDE13.pdf
・機構シミュレータMecha Mania 機構学習用テキスト
https://kano.arkoak.com/material/mechanism.pdf
・Processingでリンク機構
https://note.com/ryotako/n/n1d0a6bef62c0
コメント